The article describes how to add and configure a paragraph.
A paragraph is the main element of document content included in a section, a repeating area, and table cells. A section, a repeating area, and a table cell can contain as many paragraphs as you need. Paragraphs are used to generate text of different styles and can include URLs, inline images, page number and page count fields, and tabulations. See the Adding Content to Paragraph article for more details.
You can add paragraphs and add content to them using methods of ParagraphExtensions
and
configure paragraph settings by methods of ParagraphBuilder
.
Each paragraph has the following formatting:
Parameter |
Default value |
Methods for configuration |
Font |
|
|
Strikethrough |
|
|
Underline |
|
|
Indent for the first line of the paragraph |
No indent |
|
Line spacing |
|
|
Alignment |
|
|
Text direction |
Left to right |
|
Background color |
No color |
|
Borders |
No borders ( |
|
Margins - additional margins for a paragraph |
No additional margins |
|
Widow and orphan control |
No control |
|
Text overflow action |
|
|
URL color |
|
|
Paragraph as a list item |
|
See the Creating Multilevel List article for details on how to create and configure a list. |
Page break |
No page break |
|
Keeping with the next element |
|
Widow and Orphan Control
When you have a paragraph that starts on one page and continues to another one, the last line of the paragraph can appear at the top or bottom of a page. To prevent this, you can set the widow and orphan minimum values for your paragraphs. Setting the orphan minimum value allows you to specify the minimum number of lines that should appear at the top of the page; setting the widow minimum value allows you to specify the minimum number of lines that should appear at the bottom of the page. See Examples 7 and 8 below for code illustrations.
Page Break
When you need to start a paragraph from a new page, insert a page break before the paragraph using the method SetPageBreak()
.
If you call this method without the parameter, the paragraph will start from the next page or remain on the current page if it is located at the beginning of the page.
You can specify the page from which the paragraph will start in the method parameter. The available options are:
NextPage (the default page break) - the paragraph starts from the next page or remains on the current page if it is located at the beginning of the page.
NextEvenPage - the paragraph starts from the next even page or remains on the current page if it is located at the beginning of an even page.
NextOddPage - the paragraph starts from the next odd page or remains on the current page if it is located at the beginning of an odd page.
The available page breaks are listed in the PageBreak
enumeration.
See Example 10 below for a code illustration.
Getting Text Width
When adding a paragraph, you may need to know the actual width of a text in pixels,
for example, to calculate the proper width of the cell where this text will be inserted.
To measure the width of a text with a certain font, call the TextUtils.MeasureTextWidth
method passing the text and the font in its parameters.
For an illustration of using the method in the code of a document, see Example 11 below.
Paragraphs as TOC Items
If you are going to add a table of content (TOC) to your document, you can mark the necessary paragraphs as TOC items calling the method
ParagraphBuilder.SetOutline
. For details on how to create and configure a TOC, see the
Creating Table of Content article.
If you do not specify formatting settings for a paragraph, they will have their default values defined by the default paragraph style.
You can create a style and apply it to all paragraphs on the document, section, repeating area or table cell level using the method SetParagraphStyle
and to particular paragraphs using the method ApplyStyle
. A style can be also applied to paragraph content items (inline images, URLs, text elements, page numbers).
See Example 9 below for a code illustration. For more on working with styles, see the article Formatting and Styles.
See also
Examples
Example 1. Add and configure a paragraph with text content and borders (variant 1) Hide
DocumentBuilder.New()
.AddSection()
//Add a paragraph to the section:
.AddParagraph().SetAlignment(HorizontalAlignment.Center)
.SetFontColor(Color.Red)
.SetBorder(Stroke.Dotted)
.AddTextToParagraph("Lorem ipsum dolor sit amet")
.ToDocument().Build("Result.pdf");
The above code will generate the following:
See the document
Example 2. Add and configure a paragraph with text content and borders (variant 2) Show
Example 3. Add a paragraph with text content and background color to a header Hide
DocumentBuilder.New()
.AddSection()
//Add a header to the section:
.AddHeaderToBothPages(200)
//Add a paragraph to the header:
.AddParagraph(paragraphBuilder =>
paragraphBuilder
.SetAlignment(HorizontalAlignment.Center)
.SetFontColor(Color.Blue)
.SetFontSize(16)
.SetBackColor(Color.Yellow)
.AddText("Lorem ipsum dolor sit amet"))
.ToDocument().Build("Result.pdf");
The above code will generate the following:
See the document
Example 4. Add a paragraph with borders and margins to a repeating area by coordinates Hide
var styleParagraph = StyleBuilder.New().SetFontColor(Color.Blue);
DocumentBuilder.New()
.AddSection()
//Set the style for paragraphs:
.SetParagraphStyle(styleParagraph)
//Add a repeating area to the section:
.AddRptAreaToBothPages(0, 0, 140, 50)
//Add paragraph to header:
.AddParagraph(paragraphBuilder =>
paragraphBuilder
.SetMarginRight(20)
.SetBorder(Stroke.Dotted, Color.Red, 0.4f)
.SetFontName(FontNames.Times)
.SetAlignment(HorizontalAlignment.Right)
.AddText("Paragraph with border and margin 20"))
.ToSection()
//Add a document flow area:
.AddDocumentFlowAreaToBothPages(140, 0, 612, 50)
.AddParagraph("Document with repeating area")
.SetBorder(Stroke.Dotted, Color.Green, 0.4f)
.SetAlignment(HorizontalAlignment.Center)
.ToDocument().Build("Result.pdf");
The above code will generate the following:
See the document
Example 5. Add a paragraph to a table cell Hide
DocumentBuilder.New()
//Add a section and section content:
.AddSection()
.AddTable()
.AddColumnToTable()
.AddRow()
.AddCell()
.AddParagraphToCell("Paragraph in table cell.")
.ToDocument().Build("Result.pdf");
The above code will generate the following:
See the document
Example 6. Create a paragraph with a first line indent Hide
DocumentBuilder.New()
.AddSection()
.SetOrientation(PageOrientation.Portrait)
//Add a paragraph to the section:
.AddParagraph("This is a simple example of a first line indented paragraph. It is a common practice to indent the first line of a paragraph.")
.SetFirstLineIndent(15)
.ToDocument().Build("Result.pdf");
The above code will generate the following:
See the document
Example 7. Add a paragraph with the orphan control Hide
DocumentBuilder.New()
.AddSection()
.SetSize(150, 60)
.SetMargins(0)
.AddLineToSection(line => line.SetMarginTop(35))
//Add a paragraph to the section:
.AddParagraph("This paragraph consists of " +
"multiple lines. There should " +
"be at least two lines at the end of " +
"a paragraph on one page.")
.SetOrphanMinimum(2)
.SetWidowMinimum(0)
.ToDocument().Build("Result.pdf");
The above code will generate the following:
See the document
Example 8. Add a paragraph with the widow control Hide
DocumentBuilder.New()
.AddSection()
.SetSize(150, 60)
.SetMargins(0)
.AddLineToSection(line => line.SetMarginTop(35))
//Add a paragraph to the section:
.AddParagraph("This paragraph consists of " +
"multiple lines. There must " +
"be at least two lines at the start of " +
"a paragraph on one page.")
.SetOrphanMinimum(0)
.SetWidowMinimum(2)
.ToDocument().Build("Result.pdf");
The above code will generate the following:
See the document
Example 9. Add a paragraph with the border set in a style Hide
var styleMain = StyleBuilder.New()
.SetBorderColor(Color.Blue)
.SetBorderWidth(0.75f);
var styleParagraph = StyleBuilder.New(styleMain)
.SetBorder(Inherit.Parent)
.SetBorderStroke(Stroke.Solid);
//Create a document:
DocumentBuilder.New()
.AddSection().SetParagraphStyle(styleParagraph)
.AddParagraph("Information note")
.ToDocument().Build("Result.pdf");
The above code will generate the following:
See the document
Example 10. Add paragraphs with page breaks Hide
.AddSection()
.SetSize(PaperSize.C6)
.AddParagraph("Paragraph1 has to be left at the same page")
.SetPageBreak(PageBreak.NextPage)
.ToSection()
.AddParagraph("Paragraph2 has to be started from page #3")
.SetPageBreak(PageBreak.NextOddPage)
.ToSection()
.AddParagraph("Paragraph3 has to be started from page #4")
.SetPageBreak(PageBreak.NextEvenPage)
.ToSection()
.AddFooterToBothPages(20)
.AddParagraph()
.AddPageNumber("Page #")
.ToDocument()
The above code will generate the following:
See the document
Example 11. Getting the width of text in the cell and using it to calculate the cell width Hide
// First, we get the pixel width of the text in the cell,
// then we use this width to calculate the column width (taking into account the cell borders)
// In this case, the column width will exactly fit the text
string textInColumn1 = "Column1";
string textInColumn2 = "And this is column2";
FontBuilder font = StyleSheet.DefaultFont();
float borderWidth = StyleSheet.DefaultBorderWidth;
float width1 = TextUtils.MeasureTextWidth(textInColumn1, font);
float width2 = TextUtils.MeasureTextWidth(textInColumn2, font);
float spaceBeforeTextInCell = 0.5f;
builder
.AddSection()
.AddTable()
.AddColumnToTable("", width1 + borderWidth*2 + spaceBeforeTextInCell)
.AddColumnToTable("", width2 + borderWidth*2 + spaceBeforeTextInCell)
.AddRow()
.SetFont(font)
.AddCellToRow(textInColumn1)
.AddCellToRow(textInColumn2)
.ToDocument()
.Build("Result.pdf");
The above code will generate the following:
See the document
Example 12. Keeping a paragraph with the paragraph following it Hide
DocumentBuilder.New()
.AddSection()
.SetMargins(0)
.AddParagraph("PARAGRAPH 1")
.SetMarginTop(580)
.ToSection()
.AddParagraph("PARAGRAPH 2")
.SetKeepWithNext(true)
.ToSection()
.AddParagraphToSection("PARAGRAPH 3")
.AddParagraphToSection("PARAGRAPH 4")
.ToDocument()
.Build("Result.pdf");
The above code will generate the following:
See the document
If you use .SetKeepWithNext(false) for the same paragraph, the document will look as follows:
Example 13. Keeping a paragraph with the table following it Hide
SectionBuilder s = builder.AddSection().SetMargins(0);
for (int tableCount = 1; tableCount <= 3; tableCount++)
{
s.AddParagraph("Table " + tableCount.ToString())
.SetAlignment(HorizontalAlignment.Center).SetBold().SetFontSize(20)
.SetKeepWithNext(true);
TableBuilder t = s.AddTable().AddColumnToTable("Column 1").AddColumnToTable("Column 2");
for (int rowCount = 1; rowCount <= 16; rowCount++)
t.AddRow().AddCellToRow("Cell 1").AddCell("Cell 2");
}
s.ToDocument()
.Build("Result.pdf");
The above code will generate the following:
See the document
If you use .SetKeepWithNext(false) for the same paragraph, the document will look as follows:
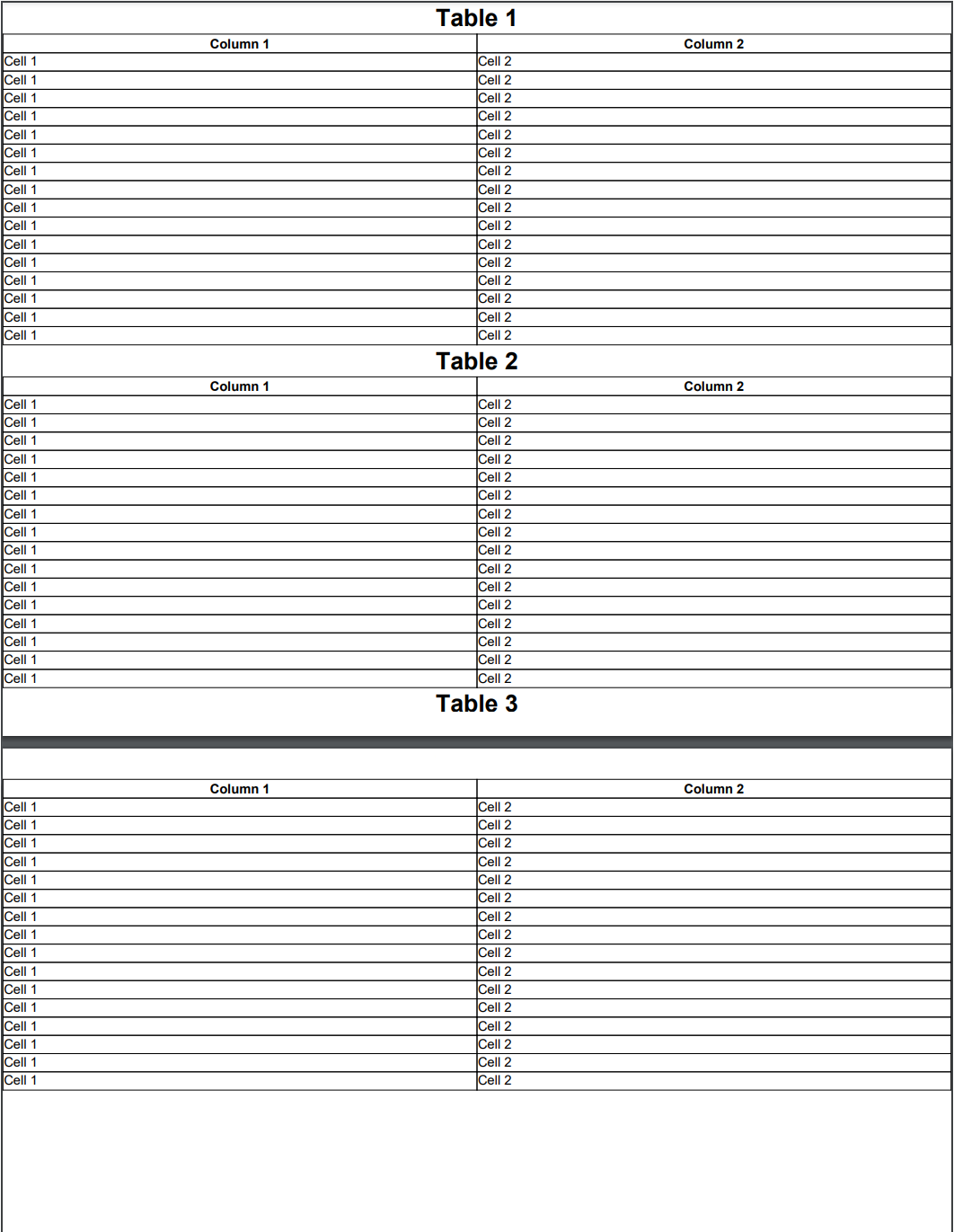